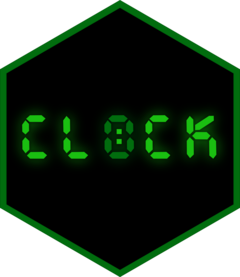
Package index
High Level API
This section contains the high level API for R’s native date and date-time types (Date, POSIXct, and POSIXlt). For most clock users, these are the only functions you’ll need.
-
date_build()
- Building: date
-
date_time_build()
- Building: date-time
-
date_today()
date_now()
- Current date and date-time
-
date_group()
- Group date and date-time components
-
date_count_between()
- Counting: date and date-time
-
date_start()
date_end()
- Boundaries: date and date-time
-
date_floor()
date_ceiling()
date_round()
- Date and date-time rounding
-
date_shift()
- Shifting: date and date-time
-
date_seq()
- Sequences: date and date-time
-
date_spanning_seq()
- Spanning sequence: date and date-time
-
date_weekday_factor()
- Convert a date or date-time to a weekday factor
-
date_month_factor()
- Convert a date or date-time to an ordered factor of month names
-
date_leap_year()
- Is the year a leap year?
-
date_time_zone()
date_time_set_zone()
- Get or set the time zone
-
date_time_info()
- Info: date-time
-
get_year(<Date>)
get_month(<Date>)
get_day(<Date>)
- Getters: date
-
get_year(<POSIXt>)
get_month(<POSIXt>)
get_day(<POSIXt>)
get_hour(<POSIXt>)
get_minute(<POSIXt>)
get_second(<POSIXt>)
- Getters: date-time
-
set_year(<Date>)
set_month(<Date>)
set_day(<Date>)
- Setters: date
-
date_format()
- Formatting: date and date-time
-
date_parse()
- Parsing: date
-
as_date()
- Convert to a date
-
as_date_time()
- Convert to a date-time
Calendars
This section contains the overarching calendar_*()
API that applies to all calendars. See the subsections for details about a specific calendar.
-
calendar_group()
- Group calendar components
-
calendar_count_between()
- Counting: calendars
-
calendar_start()
calendar_end()
- Boundaries: calendars
-
calendar_narrow()
- Narrow a calendar to a less precise precision
-
calendar_widen()
- Widen a calendar to a more precise precision
-
calendar_spanning_seq()
- Spanning sequence: calendars
-
calendar_precision()
- Precision: calendar
-
calendar_leap_year()
- Is the calendar year a leap year?
-
calendar_month_factor()
- Convert a calendar to an ordered factor of month names
-
year_month_day()
- Calendar: year-month-day
-
as_year_month_day()
- Convert to year-month-day
-
is_year_month_day()
- Is
x
a year-month-day?
-
year_month_day_parse()
- Parsing: year-month-day
-
calendar_group(<clock_year_month_day>)
- Grouping: year-month-day
-
calendar_count_between(<clock_year_month_day>)
- Counting: year-month-day
-
calendar_start(<clock_year_month_day>)
calendar_end(<clock_year_month_day>)
- Boundaries: year-month-day
-
calendar_narrow(<clock_year_month_day>)
- Narrow: year-month-day
-
calendar_widen(<clock_year_month_day>)
- Widen: year-month-day
-
seq(<clock_year_month_day>)
- Sequences: year-month-day
-
add_years(<clock_year_month_day>)
add_quarters(<clock_year_month_day>)
add_months(<clock_year_month_day>)
- Arithmetic: year-month-day
-
get_year(<clock_year_month_day>)
get_month(<clock_year_month_day>)
get_day(<clock_year_month_day>)
get_hour(<clock_year_month_day>)
get_minute(<clock_year_month_day>)
get_second(<clock_year_month_day>)
get_millisecond(<clock_year_month_day>)
get_microsecond(<clock_year_month_day>)
get_nanosecond(<clock_year_month_day>)
- Getters: year-month-day
-
set_year(<clock_year_month_day>)
set_month(<clock_year_month_day>)
set_day(<clock_year_month_day>)
set_hour(<clock_year_month_day>)
set_minute(<clock_year_month_day>)
set_second(<clock_year_month_day>)
set_millisecond(<clock_year_month_day>)
set_microsecond(<clock_year_month_day>)
set_nanosecond(<clock_year_month_day>)
- Setters: year-month-day
-
year_month_weekday()
- Calendar: year-month-weekday
-
as_year_month_weekday()
- Convert to year-month-weekday
-
is_year_month_weekday()
- Is
x
a year-month-weekday?
-
calendar_group(<clock_year_month_weekday>)
- Grouping: year-month-weekday
-
calendar_count_between(<clock_year_month_weekday>)
- Counting: year-month-weekday
-
calendar_start(<clock_year_month_weekday>)
calendar_end(<clock_year_month_weekday>)
- Boundaries: year-month-weekday
-
calendar_narrow(<clock_year_month_weekday>)
- Narrow: year-month-weekday
-
calendar_widen(<clock_year_month_weekday>)
- Widen: year-month-weekday
-
seq(<clock_year_month_weekday>)
- Sequences: year-month-weekday
-
add_years(<clock_year_month_weekday>)
add_quarters(<clock_year_month_weekday>)
add_months(<clock_year_month_weekday>)
- Arithmetic: year-month-weekday
-
get_year(<clock_year_month_weekday>)
get_month(<clock_year_month_weekday>)
get_day(<clock_year_month_weekday>)
get_index(<clock_year_month_weekday>)
get_hour(<clock_year_month_weekday>)
get_minute(<clock_year_month_weekday>)
get_second(<clock_year_month_weekday>)
get_millisecond(<clock_year_month_weekday>)
get_microsecond(<clock_year_month_weekday>)
get_nanosecond(<clock_year_month_weekday>)
- Getters: year-month-weekday
-
set_year(<clock_year_month_weekday>)
set_month(<clock_year_month_weekday>)
set_day(<clock_year_month_weekday>)
set_index(<clock_year_month_weekday>)
set_hour(<clock_year_month_weekday>)
set_minute(<clock_year_month_weekday>)
set_second(<clock_year_month_weekday>)
set_millisecond(<clock_year_month_weekday>)
set_microsecond(<clock_year_month_weekday>)
set_nanosecond(<clock_year_month_weekday>)
- Setters: year-month-weekday
-
year_week_day()
- Calendar: year-week-day
-
as_year_week_day()
- Convert to year-week-day
-
is_year_week_day()
- Is
x
a year-week-day?
-
calendar_group(<clock_year_week_day>)
- Grouping: year-week-day
-
calendar_count_between(<clock_year_week_day>)
- Counting: year-week-day
-
calendar_start(<clock_year_week_day>)
calendar_end(<clock_year_week_day>)
- Boundaries: year-week-day
-
calendar_narrow(<clock_year_week_day>)
- Narrow: year-week-day
-
calendar_widen(<clock_year_week_day>)
- Widen: year-week-day
-
seq(<clock_year_week_day>)
- Sequences: year-week-day
-
add_years(<clock_year_week_day>)
- Arithmetic: year-week-day
-
get_year(<clock_year_week_day>)
get_week(<clock_year_week_day>)
get_day(<clock_year_week_day>)
get_hour(<clock_year_week_day>)
get_minute(<clock_year_week_day>)
get_second(<clock_year_week_day>)
get_millisecond(<clock_year_week_day>)
get_microsecond(<clock_year_week_day>)
get_nanosecond(<clock_year_week_day>)
- Getters: year-week-day
-
set_year(<clock_year_week_day>)
set_week(<clock_year_week_day>)
set_day(<clock_year_week_day>)
set_hour(<clock_year_week_day>)
set_minute(<clock_year_week_day>)
set_second(<clock_year_week_day>)
set_millisecond(<clock_year_week_day>)
set_microsecond(<clock_year_week_day>)
set_nanosecond(<clock_year_week_day>)
- Setters: year-week-day
-
iso_year_week_day()
- Calendar: iso-year-week-day
-
as_iso_year_week_day()
- Convert to iso-year-week-day
-
is_iso_year_week_day()
- Is
x
a iso-year-week-day?
-
calendar_group(<clock_iso_year_week_day>)
- Grouping: iso-year-week-day
-
calendar_count_between(<clock_iso_year_week_day>)
- Counting: iso-year-week-day
-
calendar_start(<clock_iso_year_week_day>)
calendar_end(<clock_iso_year_week_day>)
- Boundaries: iso-year-week-day
-
calendar_narrow(<clock_iso_year_week_day>)
- Narrow: iso-year-week-day
-
calendar_widen(<clock_iso_year_week_day>)
- Widen: iso-year-week-day
-
seq(<clock_iso_year_week_day>)
- Sequences: iso-year-week-day
-
add_years(<clock_iso_year_week_day>)
- Arithmetic: iso-year-week-day
-
get_year(<clock_iso_year_week_day>)
get_week(<clock_iso_year_week_day>)
get_day(<clock_iso_year_week_day>)
get_hour(<clock_iso_year_week_day>)
get_minute(<clock_iso_year_week_day>)
get_second(<clock_iso_year_week_day>)
get_millisecond(<clock_iso_year_week_day>)
get_microsecond(<clock_iso_year_week_day>)
get_nanosecond(<clock_iso_year_week_day>)
- Getters: iso-year-week-day
-
set_year(<clock_iso_year_week_day>)
set_week(<clock_iso_year_week_day>)
set_day(<clock_iso_year_week_day>)
set_hour(<clock_iso_year_week_day>)
set_minute(<clock_iso_year_week_day>)
set_second(<clock_iso_year_week_day>)
set_millisecond(<clock_iso_year_week_day>)
set_microsecond(<clock_iso_year_week_day>)
set_nanosecond(<clock_iso_year_week_day>)
- Setters: iso-year-week-day
-
year_quarter_day()
- Calendar: year-quarter-day
-
as_year_quarter_day()
- Convert to year-quarter-day
-
is_year_quarter_day()
- Is
x
a year-quarter-day?
-
calendar_group(<clock_year_quarter_day>)
- Grouping: year-quarter-day
-
calendar_count_between(<clock_year_quarter_day>)
- Counting: year-quarter-day
-
calendar_start(<clock_year_quarter_day>)
calendar_end(<clock_year_quarter_day>)
- Boundaries: year-quarter-day
-
calendar_narrow(<clock_year_quarter_day>)
- Narrow: year-quarter-day
-
calendar_widen(<clock_year_quarter_day>)
- Widen: year-quarter-day
-
seq(<clock_year_quarter_day>)
- Sequences: year-quarter-day
-
add_years(<clock_year_quarter_day>)
add_quarters(<clock_year_quarter_day>)
- Arithmetic: year-quarter-day
-
get_year(<clock_year_quarter_day>)
get_quarter(<clock_year_quarter_day>)
get_day(<clock_year_quarter_day>)
get_hour(<clock_year_quarter_day>)
get_minute(<clock_year_quarter_day>)
get_second(<clock_year_quarter_day>)
get_millisecond(<clock_year_quarter_day>)
get_microsecond(<clock_year_quarter_day>)
get_nanosecond(<clock_year_quarter_day>)
- Getters: year-quarter-day
-
set_year(<clock_year_quarter_day>)
set_quarter(<clock_year_quarter_day>)
set_day(<clock_year_quarter_day>)
set_hour(<clock_year_quarter_day>)
set_minute(<clock_year_quarter_day>)
set_second(<clock_year_quarter_day>)
set_millisecond(<clock_year_quarter_day>)
set_microsecond(<clock_year_quarter_day>)
set_nanosecond(<clock_year_quarter_day>)
- Setters: year-quarter-day
-
year_day()
- Calendar: year-day
-
as_year_day()
- Convert to year-day
-
is_year_day()
- Is
x
a year-day?
-
calendar_group(<clock_year_day>)
- Grouping: year-day
-
calendar_count_between(<clock_year_day>)
- Counting: year-day
-
calendar_start(<clock_year_day>)
calendar_end(<clock_year_day>)
- Boundaries: year-day
-
calendar_narrow(<clock_year_day>)
- Narrow: year-day
-
calendar_widen(<clock_year_day>)
- Widen: year-day
-
seq(<clock_year_day>)
- Sequences: year-day
-
add_years(<clock_year_day>)
- Arithmetic: year-day
-
invalid_detect()
invalid_any()
invalid_count()
invalid_remove()
invalid_resolve()
- Invalid calendar dates
-
duration_years()
duration_quarters()
duration_months()
duration_weeks()
duration_days()
duration_hours()
duration_minutes()
duration_seconds()
duration_milliseconds()
duration_microseconds()
duration_nanoseconds()
- Construct a duration
-
as_duration()
- Convert to a duration
-
is_duration()
- Is
x
a duration?
-
duration_cast()
- Cast a duration between precisions
-
duration_floor()
duration_ceiling()
duration_round()
- Duration rounding
-
duration_precision()
- Precision: duration
-
duration_spanning_seq()
- Spanning sequence: duration
-
seq(<clock_duration>)
- Sequences: duration
-
add_years(<clock_duration>)
add_quarters(<clock_duration>)
add_months(<clock_duration>)
add_weeks(<clock_duration>)
add_days(<clock_duration>)
add_hours(<clock_duration>)
add_minutes(<clock_duration>)
add_seconds(<clock_duration>)
add_milliseconds(<clock_duration>)
add_microseconds(<clock_duration>)
add_nanoseconds(<clock_duration>)
- Arithmetic: duration
-
time_point_cast()
- Cast a time point between precisions
-
time_point_count_between()
- Counting: time point
-
time_point_floor()
time_point_ceiling()
time_point_round()
- Time point rounding
-
time_point_shift()
- Shifting: time point
-
time_point_precision()
- Precision: time point
-
time_point_spanning_seq()
- Spanning sequence: time points
-
seq(<clock_time_point>)
- Sequences: time points
-
as_sys_time()
- Convert to a sys-time
-
is_sys_time()
- Is
x
a sys-time?
-
sys_time_parse()
sys_time_parse_RFC_3339()
- Parsing: sys-time
-
sys_time_info()
- Info: sys-time
-
sys_time_now()
- What is the current sys-time?
-
as_zoned_time(<clock_sys_time>)
- Convert to a zoned-time from a sys-time
-
as_naive_time()
- Convert to a naive-time
-
is_naive_time()
- Is
x
a naive-time?
-
naive_time_parse()
- Parsing: naive-time
-
naive_time_info()
- Info: naive-time
-
as_zoned_time(<clock_naive_time>)
- Convert to a zoned-time from a naive-time
-
as_zoned_time()
- Convert to a zoned-time
-
is_zoned_time()
- Is
x
a zoned-time?
-
zoned_time_parse_complete()
zoned_time_parse_abbrev()
- Parsing: zoned-time
-
zoned_time_now()
- What is the current zoned-time?
-
zoned_time_zone()
zoned_time_set_zone()
- Get or set the time zone
-
zoned_time_precision()
- Precision: zoned-time
-
zoned_time_info()
- Info: zoned-time
-
format(<clock_zoned_time>)
- Formatting: zoned-time
-
weekday()
- Construct a weekday vector
-
as_weekday()
- Convert to a weekday
-
is_weekday()
- Is
x
a weekday?
-
weekday_code()
- Extract underlying weekday codes
-
weekday_factor()
- Convert a weekday to an ordered factor
-
add_days(<clock_weekday>)
- Arithmetic: weekday
-
clock_locale()
- Create a clock locale
-
clock_labels()
clock_labels_lookup()
clock_labels_languages()
- Create or retrieve date related labels
-
clock_months
clock_weekdays
clock_iso_weekdays
- Integer codes
-
vec_arith(<clock_year_day>)
vec_arith(<clock_year_month_day>)
vec_arith(<clock_year_month_weekday>)
vec_arith(<clock_iso_year_week_day>)
vec_arith(<clock_naive_time>)
vec_arith(<clock_year_quarter_day>)
vec_arith(<clock_sys_time>)
vec_arith(<clock_year_week_day>)
vec_arith(<clock_weekday>)
- Support for vctrs arithmetic
Generics and methods
This section contains documentation about generics and methods that is already exposed elsewhere on this reference page.
-
add_years()
add_quarters()
add_months()
add_weeks()
add_days()
add_hours()
add_minutes()
add_seconds()
add_milliseconds()
add_microseconds()
add_nanoseconds()
- Clock arithmetic
-
get_year()
get_quarter()
get_month()
get_week()
get_day()
get_hour()
get_minute()
get_second()
get_millisecond()
get_microsecond()
get_nanosecond()
get_index()
- Calendar getters
-
set_year()
set_quarter()
set_month()
set_week()
set_day()
set_hour()
set_minute()
set_second()
set_millisecond()
set_microsecond()
set_nanosecond()
set_index()
- Calendar setters
-
date_group(<Date>)
- Group date components
-
date_group(<POSIXt>)
- Group date-time components
-
date_count_between(<Date>)
- Counting: date
-
date_count_between(<POSIXt>)
- Counting: date-times
-
date_start(<Date>)
date_end(<Date>)
- Boundaries: date
-
date_start(<POSIXt>)
date_end(<POSIXt>)
- Boundaries: date-time
-
date_format(<Date>)
- Formatting: date
-
date_format(<POSIXt>)
- Formatting: date-time
-
date_floor(<Date>)
date_ceiling(<Date>)
date_round(<Date>)
- Rounding: date
-
date_floor(<POSIXt>)
date_ceiling(<POSIXt>)
date_round(<POSIXt>)
- Rounding: date-time
-
date_shift(<Date>)
- Shifting: date
-
date_shift(<POSIXt>)
- Shifting: date and date-time
-
date_seq(<Date>)
- Sequences: date
-
date_seq(<POSIXt>)
- Sequences: date-time
-
as_zoned_time(<Date>)
- Convert to a zoned-time from a date
-
as_zoned_time(<POSIXt>)
- Convert to a zoned-time from a date-time
-
reexports
tzdb_names
tzdb_version
- Objects exported from other packages